Data types #
Data types define the type of variable, constant, function argument and the value returned by a function. For example, if a variable is defined as Numeric, it will contain a number, if defined as String then it will contain a string of characters etc.
Data type is defined using AS keyword followed by the data type:
Dim b As Numeric
Data types available in Overbasic are:
Numeric: represents a number. In OverBasic there is no distinction between integers, decimals, etc. Decimal separator is a point “.”
Boolean: represents a Boolean value (TRUE or FALSE)
String: represents a string of characters
Database: represents the data series of an instrument
TradingSystem: represents a Trading System
Date: (available ONLY in PROPERTY) represents a date in the YYYYMMDD format
Time: (available ONLY in PROPERTY) represents a time in the HHMMSSFFFCCCN format (HH = hour; MM = minutes; SS = seconds; FFF = milliseconds; CCC = microseconds; N = 100 nanoseconds)
Indicator: (available ONLY in PROPERTY) represents an indicator.
Variable #
A variable is an object containing data (a number, boolean, string, etc.). Variables can be used in any expression in the Overbasic script (within variable scope).
Syntax #
Before being used, a variable must be declared, that is, Overbasic must be informed of its existence and the type of data it can contain. Variable declaration is done using DIM keyword followed by the variable name, data type and initialization value/expression (optional):
Dim <variable name> As <data type>[()] = [<initialization value/expression>]
Variable name:
- must be unique within the script
- can be any string of letters and numbers
- CANNOT be an Overbasic keyword, or the name of a function, argument, etc.
Dim a As Numeric = 10
'10 is the initialization value
Array:
An array is an ordered collection (a series) of items of the same type. Access to an array item is via its numeric index. The first item of an array always has index 0 (zero).
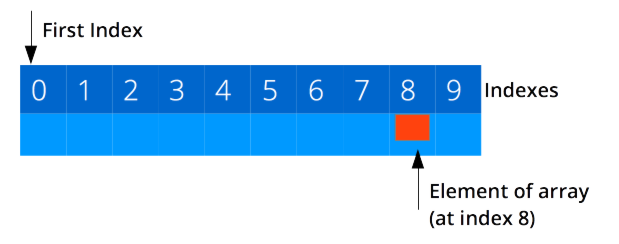
A variable can be declared as an array by adding round brackets to the data type:
Dim a As Numeric()
An array can be initialized in 2 different ways:
By item:
'Each array item is initialized to 10.
Dim a As Numeric() = 10
With another array:
Dim a1 As Numeric() = 10
a1(5) = 23 'let's use a1 at least once before assigning it to a2
Dim a2 As Numeric() = a1
Array a2 is initialized with array a1. In this case it is not an initialization, but an assignment by reference. In other words, a1 and a2 actually represent the same object and therefore a subsequent modification to an element of a1 will be reflected on the same element of a2. The above is valid ONLY if a1 has been used at least once before being assigned to a2. This is because arrays in Overbasic are created and therefore only really exist when they are first used.
It is not necessary to define the array size. The size is automatically managed by Overbasic.
Access to an array item is via its numeric index. The first item of an array always has index 0 (zero):
Dim a1 As Numeric() = 10
a1(5) = 23 'this line assigns the value 23 to item 5 of array a1
To delete all array items, use ERASE keyword:
Erase a1
Constant #
A constant is an object containing data that does not change during script execution.
Syntax #
Before being used, a constant must be declared, i.e. Overbasic must be informed of its existence, data type and value. Constant declaration is done using CONST keyword followed by the constant name, data type and value:
Const <constant name> As <data type> = <value/expression>
Costante name:
- must be unique within the script
- can be any string of letters and numbers
- CANNOT be an Overbasic keyword, or the name of a function, argument, etc.
Const a As Numeric = 10
Scope #
Scope is the region in which a variable/constant is valid and can be used. In other words, a variable/constant can only be used in the context in which it was declared.
If declaration is module level (outside of all functions), variable/constant is valid everywhere in the script and its value is NOT initialized every time the script is run:
Dim a As Numeric = 10
Function Main()
...
If (a = 10) Then
...
EndIf
...
EndFunction
Function CalculateABS As Numeric
If (a < 0) Then
Return -1 * a
EndIf
Return a
EndFunction
If declaration is internal to a function, variable/constant will be valid only internally to the function and only in statements following its declaration:
Function Main()
Dim a As Numeric = 10
...
If (a = 10) Then
...
EndIf
...
EndFunction
If a variable is declared inside an IF, FOR, DO UNTIL, WHILE, SELECT CASE etc, it can only be used in the context in which it was declared:
Function Main()
Dim b As Boolean = True
...
If (b = True) Then
Dim a As Numeric = 10
...
a = Close
...
EndIf
...
a = 10 * High 'ERROR! It is NOT possible to use the variable outside the IF where it was declared
...
EndFunction
Static #
A variable declared inside a function will be initialized each time the script is executed. However, in particular situations, it can be useful to keep its last value avoiding reinitialization.
To do this you have 2 possibilities:
- You declare a module-level variable (outside of all functions)
- You declare a variable within the function using STATIC keyword instead of DIM keyword:
Static n As Numeric = 0
Example:
Function Main()
...
'Initialization to 0 only occurs the first time.
'In the following runs, last previous value is kept
Static n As Numeric = 0
...
If (Open > Close) Then
n = n + 1
EndIf
...
...
Return n
EndFunction